Raycast is a popular technique to allow your program to detect mouse clicks on your game objects. For example you can use this technique to detect if your player has select which enemy to battle against.
There is a lots of video and material out there on how to implement this technique. However, most of them failed to mention 2 important points about this technique. I will let you know what are 2 important points to make this technique work.
First, the methods to use differ depending on whether you are making a 2D or a 3D game. In 2D game implementation, you can use this sample code to implement raycast.
//2D only
touchPos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
RaycastHit2D hit = Physics2D.Raycast(touchPos, Vector2.zero);
if(hit.collider != null)
{
switch (hit.collider.name)
{
case "Weapon1":
print("Weapon1 selected");
break;
case "Weapon2":
print("Weapon2 selected");
break;
case "Weapon3":
print("Weapon3 selected");
break;
}
}
If you implement this for a 3D game, the script is a bit different. Here is a sample script for 3D game implementation.
//3D only
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit3D;
if(Physics.Raycast(ray, out hit3D))
{
switch (hit3D.collider.name)
{
case "Weapon1":
print("Weapon1 selected");
break;
case "Weapon2":
print("Weapon2 selected");
break;
case "Weapon3":
print("Weapon3 selected");
break;
}
}
The second important to note and it is often left out in implementing a raycast is that the objects to be selected must have a Collider component attached to it. If it is 2D game then we will need to attach a 2D collider to our game object and a 3D collider to a 3D game object.
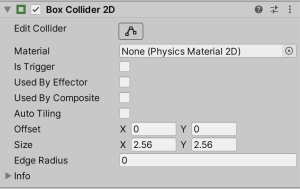
In summary, you need to remember 2 things:
- Remember to use 2D code for 2D implementation.
- Remember to attach a collider to your game objects.
Recent Comments